Lab 02
File I/O Problems
Objective:
Write a solution that performs file input and output (File
I/O) using a variety of file types and problems.
Lab Solution
Requirements:
- Functionality. (80pts)
- No Syntax, Major Run-Time, or Major Logic Errors.
(80pts*)
- *Code that cannot be compiled due to syntax errors is
nonfunctional code and will receive no points for this entire section.
- *Code that cannot be executed or tested due to major
run-time or logic errors is nonfunctional code and will receive no
points for this entire section.
- *Files used for reading and writing must use relative file
paths that reference the project’s root folder. Any use of absolute file
paths or paths that do not reference the project’s root directory will receive
no points for this entire section.
- Set up the Project. (20pts)
- First download the driver file and the text
files (ItIs.txt and Tubes.txt)and include it in
your project.
- Create a class and name it, exactly, FileIOSolutions.
- Do not modify the provided driver or text file.
- All must apply for full credit.
- Write method pastTense. (30pts)
- This static method returns no values and is provided
both with a read file name and an output file name.
- The provided read file is assumed to be plain text and
each word is separated using either spaces, tabs, or end-line
characters.
- The method reads the provided file word-by-word
and changes every instance of “is” to “was” and case does not matter.
- The method must both print each word to the console and
to a new file on a new line. The formatting in the read file can be ignored,
such as spaces, tabs and end-lines.
- All must apply for full credit.
- Write method totalTubeVolume. (30pts)
- This static method returns the total combined volumes of
tubes in a file.
- The file is assumed to be tab-delimited and has the
following format,
- <<id>>\t<<tube’s
radius>>\t<<tube’s height>>\n
…
- The method must read, parse, and process the information
in the file line-by-line to calculate the total combined volumes.
- The method should ignore any lines in the file that are not
properly formatted.
- It can be assumed that the amount of tubes in the file will
never exceed 100.
- Volume can be calculated by

- All must apply for full credit.
- Coding Style. (10pts)
- Code functionality organized within multiple methods
other than the main method, and methods organized within multiple classes
where appropriate. (5pts)
- Readable Code (5pts)
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements
within the body of a class, a method, a branching statement, a loop
statement, etc.
- All the above must apply for full credit.
- Comments. (10pts)
- Your name in the file. (5pts)
- At least 5 meaningful comments in addition to your name.
These must describe the function of the code it is near. (5pts)
Example Dialog:
Welcome to the File I/O Problem's Solutions!
Enter 1. For Test 1
Enter 2. For Test 2
Enter 9. To Quit
1
-----------------------
Test01
-----------------------
Testing method "pastTense" with the file
"ItIs.txt" and outputting to "ItWas.txt"
What
was
it?
It
was
something
after
all,
and
something
was
not
nothing.
How
was
it
described?
was
it
big?
was
it
small?
was
it
green
or
was
it
purple
or
was
it
neither?
was
this
it
or
was
that
it?
Who
knows?
Enter 1. For Test 1
Enter 2. For Test 2
Enter 9. To Quit
2
-----------------------
Test02
-----------------------
Testing method "totalTubeVolume" with the file
"./Tubes.txt"
The total combined volume is: 20106.51499622167
Enter 1. For Test 1
Enter 2. For Test 2
Enter 9. To Quit
9
Goodbye!
Solution Tests:
- Is your name written as a comment in all source files?
- Does the solution compile (no syntax errors)?
- When running the driver, do you get the same output as the
Example Dialog?
Lab Report
- Create a section named “Problem” and describe this lab’s
problem in your own words. (10pts).
- Create a section named “Solution Description” and describe
how the code solves the problem in your own words. (10pts).
- Create a section named “Problems Encountered” and describe
the various syntax, run-time, and logic errors that were encountered while
implementing the solution. (10pts).
- Describe the difference between absolute and relative file
paths (10pts)
- In terms of file paths, what does “./” mean? (10pts)
- In this course, where should all files that are to be read
from or printed to be placed? (10pts)
- What important detail must one do when finished either
reading from or writing to a file? (10pts)
- Describe the purpose of “delimiters / separators” used in
file formats. (10pts)
- Looking at the below code snippet, it is supposed to read
a tab-delimited file, parse the information, and process the data. The
method “process” works correctly, but there is something wrong with how
the file is being read. It seems to read it word-by-word instead of
line-by-line, so what could be causing this problem and how can it be fixed?
(10pts)
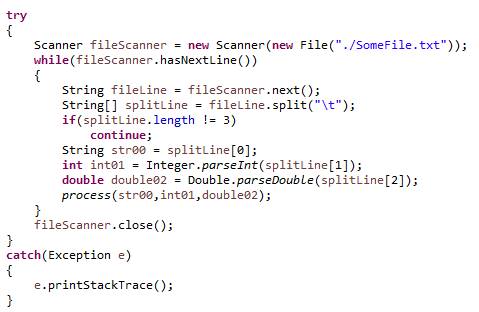
- Looking at the below code snippet, its purpose is to
append all the values in the array “someData” to the file “output.txt”.
However, this code does not append to the file, but rather erases and overwrites
all the data previously in the file. How can this code be fixed so that
the information is appended? (10pts)
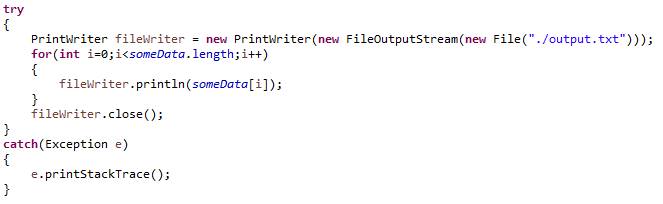
Finally
Upload the Lab Solution’s source code (.JAVA file(s)) and
the Lab Report’s text file to the CSCE Dropbox.