Lab 00
Just Follow the Instructions
Objective:
Implement bubble sort for an array of integers by following
the instructions.
Lab Solution
Requirements:
Just follow the instructions exactly to implement bubble
sort of an array of integers (100pts).
Instructions:
- In a preferred IDE,
create a project and name it “CSCE146Lab00”
- Download the provided
code from here
- Do not alter the
sections or statements that have the comments “Do not alter”
- Include the provided
code in your project’s source (SRC) folder and open it
- Change the comment
<<YOUR NAME HERE>> to your name
- Under the comment
“//Packages” include the following code
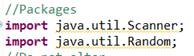
- Inside the body of the
class, but outside of the main method declare a constant named,
“ARRAY_SIZE” and set it to 10.

- Inside the main
method, create a new instance of data type Scanner for user input.
Call it keyboard and have it scan the standard system input, as follows.

- Under that statement
prompt the user for 10 numbers, by using the constant “ARRAY_SIZE”

- Declare and initialize
an array named, “array” of size 10 by using the constant “ARRAY_SIZE”

- Write a for-loop that
populates each element of the array while prompting the user.
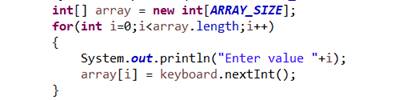
- Sort the array using
“Bubble Sort”. This algorithm examines each element of the array
side-by-side, and swaps whenever values are out of order. For instance, if
we were sorting in ascending order (smallest to largest), then if we found
at a given index that its next neighbor was smaller, then we would swap
those values. This procedure runs until no swaps are made.
- In
order to
implement this start by creating a loop that
checks if there were no swaps made. Once inside of the loop assume that no
swaps were made.
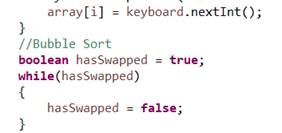
- Next, while still inside
of the while-loop write a for-loop that will check each element
side-by-side. Make sure the loop stops at “array.length-1”
to avoid index out of bounds exceptions.

- Write an if-statement
that checks the current value with its next neighbor using its indices
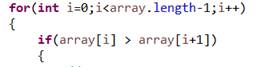
- Inside the body of the
if-statement create a temporary variable and swap the values found at
those indices (whose values were determined to be out-of-order).
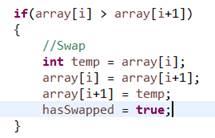
- Outside of the
while-loop but still inside of the body of the main method, write a
for-loop that prints all of the sorted values.
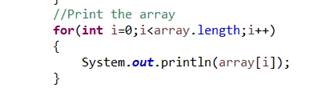
- Run the software and see
if it works!
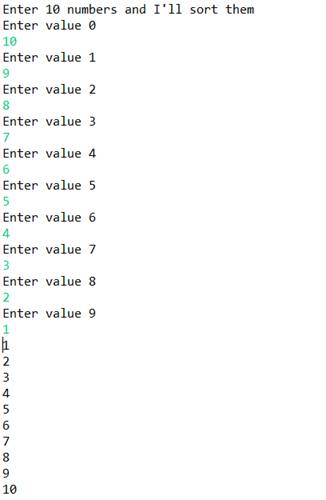
- The solution part of the
Lab is now complete. Make sure to follow the additional instructions for
testing the solution to ensure the code meets all requirements. In
addition, make sure to follow the instructions for the Lab Report and
submit both the Lab Solution and Lab Report to appropriate locations in
the CSCE Dropbox.
Solution Tests
- Does the given input
<10,9,8,7,6,5,4,3,2,1> give the following output
<1,2,3,4,5,6,7,8,9,10>?
- Does the given input
<1,2,3,4,5,6,7,8,9,10> give the following
output <1,2,3,4,5,6,7,8,9,10>?
- Does the given input
<180, 300, 256, 360, 512, 365, 7, 42, 9, 12> give the following
output <7, 9, 12, 42, 180, 256, 300, 360, 365, 512>?
Lab Report
- Describe how the course
grade is calculated. Make sure to include assignment types and their
percentages. (10pts)
- Where are all
assignments submitted and where are the grades + feedback for assignments
found? (10pts)
- What is the policy
regarding,
- Late work?
- Make-up work?
- Regrade requests?
(10pts)
- True or False. All
assignments must be completed individually. (10pts)
- For programming
assignments, what must be submitted to the CSCE Dropbox and what is its
file extension? (10pts)
- In the Java Programming
Language, when a source code is compiled using the Java Compiler, is it compile to machine code or byte code? (10pts)
- Looking at the below
code snippet, is this code error free and if so, what will it print to the
console? If the code does have errors, then describe all syntax, run-time,
and logic errors and how they may be fixed. (10pts)
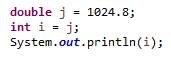
- Looking at the below
code snippet, is this code error free and if so, what will it print to the
console? If the code does have errors, then describe all syntax, run-time,
and logic errors and how they may be fixed. (10pts)
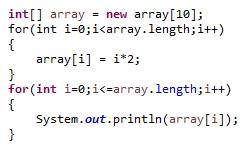
- Looking at the below
code snippet, is this code error free and if so, what will it print to the
console? If the code does have errors, then describe all syntax, run-time,
and logic errors and how they may be fixed. (10pts)
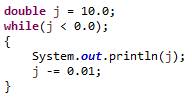
- Looking at the below
code snippet, is this code error free and if so, what will it print to the
console? If the code does have errors, then describe all syntax, run-time,
and logic errors and how they may be fixed. (10pts)
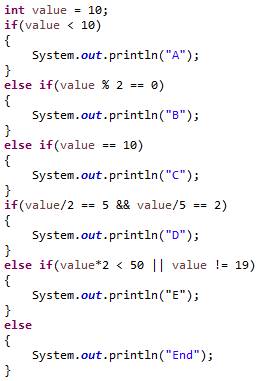
Finally:
- Upload the source code
(.JAVA File Extension) to LabSolution00
- And written lab report
(DOC, .DOCX, or .PDF file extension) to LabReport00
- To Blackboard
- Following these instructions.
- If there are problems,
then let the instructor know as soon as possible.