Homework 05
Triangles! Oh Fractals!
Due 11/15/2024 by 11:55PM
Objective:
Write a program in which it draws a triangular fractal called
Sierpinski’s Triangle using recursion and Java Swing.
Requirements:
- Functionality. (80pts)
- No Syntax, Major Run-Time, or Major Logic Errors.
(80pts*)
- *Code that cannot be compiled due to syntax errors is
nonfunctional code and will receive no points for this entire section.
- *Code that cannot be executed or tested due to major
run-time or logic errors is nonfunctional code and will receive no
points for this entire section.
- Recursive Method (50pts)
- A recursive method must be the primary way each triangle
is drawn.
- It must determine the positions of each of the 3 points
to be drawn.
- This recursive method must not be infinite and must stop
when it reaches a pixel limit of 4.
- Draw the Image (40pts)
- The fractal must be drawn using a Graphical User
Interface (GUI).
- See examples below.
- Run-Time and Logic Error Checking. (10pts)
- Each major function must check for common run-time and
logic errors.
- Coding Style. (10pts)
- Code functionality organized within multiple methods
other than the main method, and methods organized within multiple classes
where appropriate. (5pts)
- Readable Code (5pts)
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements
within the body of a class, a method, a branching statement, a loop
statement, etc.
- All the above must apply for full credit.
- Comments. (10pts)
- Your name in the file. (5pts)
- At least 5 meaningful comments in addition to your name.
These must describe the function of the code it is near. (5pts)
Suggested Methodology
The idea for it is this
- First draw a filled triangle
- Next draw another filled triangle of a different color
that’s upside down in the middle of that triangle
- Using the other triangles formed repeat step 2 until a
pixel limit of 4 is reached
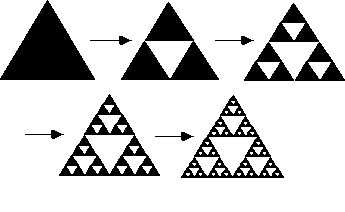
Additional Notes:
- JFrame and Canvas are two types that may help.
- The method fillPolygon(int[] xPoints, int[] yPoint,
numberOfPoints) as called by the graphics device is important
- The method setColor(Color aColor) is important for
picking different colors to draw things.
Example Image of Results:
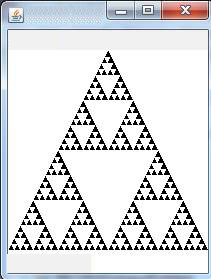
Finally:
Submit the Java Source Files (.JAVA) to the CSCE Dropbox (https://dropbox.cse.sc.edu)