Homework 00
Vector Math Program
Due 09/13/2024 by 11:55PM
Objective:
Write a program that performs a variety of vector math
operations. These operations include adding vectors, subtracting vectors, and
finding the magnitude of a vector.
Requirements:
- Functionality. (80pts)
- No Syntax, Major Run-Time, or Major Logic Errors.
(80pts*)
- *Code that cannot be compiled due to syntax errors is
nonfunctional code and will receive no points for this entire section.
- *Code that cannot be executed or tested due to major
run-time or logic errors is nonfunctional code and will receive no
points for this entire section.
- Use only Arrays to represent the Vectors and assume a
decimal type. (80pts*)
- *Other built in types like ArrayLists, LinkedLists, etc
will receive no points for this entire section.
- Clear and Easy-To-Use Interface. (10pts)
- Users should easily understand what the program does and
how to use it.
- Users should be prompted for input and should be able to
enter data easily.
- Users should be presented with output after major
functions, operations, or calculations.
- Users should be able to perform any number of the
required functions. In addition, users should be able to choose when to
terminate the program.
- All the above must apply for full credit.
- Adding Two Vectors. (20pts)
- Users should be able to enter the size and values for
both Vectors. The size of a vector must be greater or equal to 1 and the
two vectors being added must have the exact same size. Otherwise, the
user must be prompted there is an error and the program must continue
and not terminate.
- The program should clearly output the resulting Vector
to the user.
- All the above must apply for full credit.
- Subtracting Two Vectors. (20pts)
- Users should be able to enter the size and values for both
Vectors. The size of a vector must be greater or equal to 1 and the two
vectors being added must have the exact same size. Otherwise, the user
must be prompted there is an error and the program must continue and not
terminate.
- The program should clearly output the resulting Vector
to the user.
- All the above must apply for full credit.
- Find the Magnitude of a Vector. (20pts)
- Users should be able to enter the size and values of the
Vector. The size of a vector must be greater or equal to 1. Otherwise,
the user must be prompted there is an error and the program must
continue and not terminate.
- The program should clearly output the resulting
magnitude to the user.
- All the above must apply for full credit.
- Run-Time and Logic Error Checking. (10pts)
- Each major function must check for common run-time and
logic errors.
- Coding Style. (10pts)
- Code functionality organized within multiple methods
other than the main method. (5pts)
- Readable Code (5pts)
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements
within the body of a class, a method, a branching statement, a loop
statement, etc.
- All the above must apply for full credit.
- Comments. (10pts)
- Your name in the file. (5pts)
- At least 5 meaningful comments in addition to your name.
These must describe the function of the code it is near. (5pts)
Additional Notes:
- Vector Addition.
- Adding together two vectors first requires each vector
have the same number of components (the same size). The result of vector
addition creates a new vector where its components are the result of each
component-wise addition.
- Example:
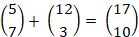
- Vector Subtraction.
- Subtracting two vectors first requires each vector have
the same number of components (the same size). The result of vector
subtraction creates a new vector where its components are the result of
each component-wise subtraction.
- Example:
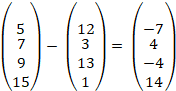
- Vector Magnitude.
- Finding the magnitude of a vector requires finding the
square root of the sum of its components squared.
- Example:
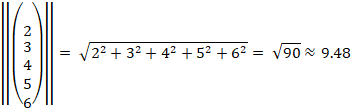
Example Dialog:
*The following Example Dialog demonstrates the interactions between a user
and ONE possible implementation of the required software’s front-end / user
interface. The software’s front-end / user interface may be implemented in MANY
different ways and will receive full credit as long as it meets the most
minimal of the above requirements. While you may use the example dialog as a
guide, it is strongly encouraged to create the front-end / user interface in
your own way. *
Key
|
Unhighlighted
Text
|
Program’s
Output
|
Highlighted
Text
|
User’s
Input
|
Welcome to the Vector Operations Program!
Enter 1. To Add 2 Vectors
Enter 2. To Subtract 2 Vectors
Enter 3. To Find the Magnitude of a Vector
Enter 9. To Quit
1
Enter the size of the Vectors
3
Enter values for Vector1
1.0
2.0
3.0
Enter values for Vector2
4.0
5.0
6.0
Result:
1.0
2.0
3.0
+
4.0
5.0
6.0
=
5.0
7.0
9.0
Enter 1. To Add 2 Vectors
Enter 2. To Subtract 2 Vectors
Enter 3. To Find the Magnitude of a Vector
Enter 9. To Quit
2
Enter the size of the Vectors
3
Enter values for Vector1
1.0
2.0
3.0
Enter values for Vector2
4.0
5.0
6.0
Result:
1.0
2.0
3.0
-
4.0
5.0
6.0
=
-3.0
-3.0
-3.0.
Enter 1. To Add 2 Vectors
Enter 2. To Subtract 2 Vectors
Enter 3. To Find the Magnitude of a Vector
Enter 9. To Quit
3
Enter the size of the Vector
5
Enter values for the Vector
1.0
2.0
3.0
4.0
5.0
The magnitude is: 7.416198487095663
Enter 1. To Add 2 Vectors
Enter 2. To Subtract 2 Vectors
Enter 3. To Find the Magnitude of a Vector
Enter 9. To Quit
2
Enter the size of the Vectors
0
Invalid Size
Enter 1. To Add 2 Vectors
Enter 2. To Subtract 2 Vectors
Enter 3. To Find the Magnitude of a Vector
Enter 9. To Quit
9
Goodbye!
Finally:
Submit the source files (.JAVA extension) to the CSCE
Dropbox (https://dropbox.cse.sc.edu)