Lab 09
Bunch of Lines
Objective:
Write a bunch of classes that will draw a bunch of
lines! Don’t worry you don’t have to write any graphics, as that part is
provided in the driver. Each line is drawn based on a math function that
takes in a given x coordinate and will return its y coordinate.
Lab Solution
- Functionality. (80pts)
- No Syntax Errors.
(80pts*)
- *Code that cannot be
compiled due to syntax errors is nonfunctional code and will receive no
points for this entire section.
- Set-Up the Project
(5pts)
- First download the driver and put it in
your project
- Do not alter the
provided code.
- Write an interface
called Line
- Method definition
- getYPoint: This takes
in a decimal value and returns a decimal value depending on the type of
line.
- Write a class
called SlopedLine
- This should implement
Line
- Instance variable
- slope: a decimal
value corresponding to the line’s slope
- Constructors
- Accessors and Mutators
for each variable
- Method
- getYPoint: this
method takes in a decimal value corresponding to a x-coordinate and
returns the y-coordinate based on the slope equation (y = slope*x)
- All must apply for
full credit.
- Write a class
called ExponentialLine
- This should implement
Line
- Instance variable
- exponent: a decimal
value corresponding to the line’s exponent
- Constructors
- Accessors and Mutators
for each variable
- Methods
- getYPoint: this
method takes in a decimal value corresponding to a x-coordinate and
returns the y-coordinate based on the slope equation (y = x^exponent)
- Write a class
called SineLine
- This should implement
Line
- Instance variables
- amplitude: a decimal
value corresponding to the line’s amplitude
- frequency: a decimal
value corresponding to the line’s frequency
- Create the following
Constructors
- Accessors and Mutators
for each variable
- Methods
- getYPoint – this
method takes in a decimal value corresponding to a x-coordinate and
returns the y-coordinate based on a sine wave equation (y =
amplitude*sin(x*frequency))
- Write a class
called SawLine
- This should implement
Line
- Instance variable
- modValue: a decimal
value corresponding to the modulo peak of the wave
- Constructors
- Accessors and Mutators
for each variable
- Methods
- getYPoint – this
method takes in a decimal value corresponding to a x-coordinate and
returns the y-coordinate based on the equation (y = x mod modValue)
- Write a class
called StaircaseLine
- This should implement
Line
- Instance variables
- width: a decimal
value corresponding to the stair’s width
- height: a decimal
value corresponding to the stair’s height
- Constructors
- Accessors and Mutators
for each variable
- Methods
- getYPoint – this
method takes in a decimal value corresponding to a x-coordinate and
returns the y-coordinate based the width and height. HINT(using integer
division and multiplying that by the height will achieve the effect).
HINT: If the lines look weird it may be a good idea
to print out each of the coordinates and observing what is going on in the
method getYPoint.
Example Dialog:
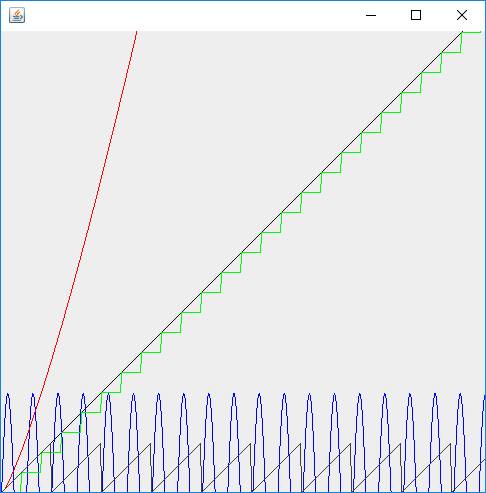
Lab Report Questions
- Draw a UML class diagram
for this project
- Describe polymorphism
Solution Tests:
- Is your name written as
a comment in all source files?
- Does the solution
compile (no syntax errors)?
3. Does your output
match the example GUI?
Lab Report
- Create a section named
“Problem” and describe this lab’s problem in your own words. (10pts).
- Create a section named
“Solution Description” and describe how the code solves the problem in
your own words. (10pts).
- Create a section named
“Problems Encountered” and describe the various syntax, run-time, and
logic errors that were encountered while implementing the solution.
(10pts).
- Describe
polymorphism in OOP. (10pts).
- Briefly
describe the similarities between classes and interfaces in Java. (10pts).
- Briefly
describe the differences between classes and interfaces in Java. (10pts).
- If we consider Classes
to be “blueprints” for Objects, then what is the relationship between
Interfaces and Classes? Interfaces and Objects? (10pts).
- Is it possible to
construct instances of an Interface in Java? (10pts).
- What
reserved word is used to establish a polymorphic relationship between a
class and an interface in Java? (10pts).
- What reserved word is
used to establish inheritance (“is a”) relationship between two interfaces
in Java? (10pts).
Finally:
- Upload the source code
(.JAVA File Extension) to LabSolution09
- And written lab report
(DOC, .DOCX, or .PDF file extension) to LabReport09
- To Blackboard
- Following these instructions.
- If there are problems,
then let the instructor know as soon as possible.