Homework 07
Cats and Dogs
Due 04/11/2025 by 11:55pm
Objective:
Write a program where a user populates
a collection of various cats and dogs. The user should be able to specify
which type they wish to enter, and then are prompted with pertinent information
for every type of pet. In addition, the solution must follow the design
provided.
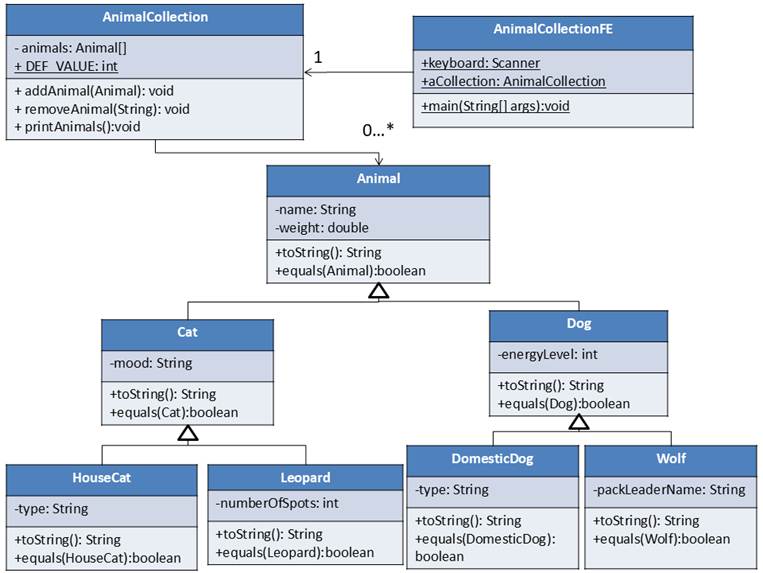
Requirements:
- Functionality. (80pts)
- No Syntax Errors. (80pts*)
- *Code that cannot be compiled due to syntax errors is
nonfunctional code and will receive no points for this entire section.
- Write a class called Animal with the following: (10pts)
- Instance Variables
- Name: The name of the animal. This value should not be
null, and its default value is “none”.
- Weight: A decimal value that must be strictly greater
than 0 and its default value is 1.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must follow
the order of the instance variables given, so first one is first,
second is second, etc. This must also check for valid values before
they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of Animal
and only returns true if all of the instance variables match. For name
and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called Cat with the following: (5pts)
- Cat must inherit from type Animal
- Instance Variables
- Mood: The cat’s mood must be either “sleepy”,
“playful”, or “hungry” and the default is “sleepy”.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must include
all the inherited values in their specified order first and then follow
the order of this class’s instance variables given, so first one is
first, second is second, etc. This must also check for valid values
before they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of Cat
and only returns true if all of the instance variables match. For name
and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called Dog with the following: (5pts)
- Cat must inherit from type Animal
- Instance Variables
- EnergyLevel: a whole number values from 0 to 100
inclusive and default value is 50.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must include
all the inherited values in their specified order first and then follow
the order of this class’s instance variables given, so first one is
first, second is second, etc. This must also check for valid values
before they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of Dog
and only returns true if all of the instance variables match. For name
and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called HouseCat with the following:
(5pts)
- Cat must inherit from type Cat
- Instance Variables
- Type: a String value that represents the House Cat’s
type. It can either be “short hair”, “ragdoll”, “sphinx”, or “Scottish
fold” and the default value is “short hair”.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must include
all the inherited values in their specified order first and then follow
the order of this class’s instance variables given, so first one is
first, second is second, etc. This must also check for valid values
before they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of House Cat
and only returns true if all of the instance variables match. For name
and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called Leopard with the following: (5pts)
- Cat must inherit from type Cat
- Instance Variables
- Number of Spots: a whole number value that must be
greater than or equal to 1. It’s default value is also 1.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must include
all the inherited values in their specified order first and then follow
the order of this class’s instance variables given, so first one is
first, second is second, etc This must also check for valid values
before they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of Leopard
and only returns true if all of the instance variables match. For name
and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called DomesticDog with the
following: (5pts)
- Cat must inherit from type Dog
- Instance Variables
- Type: a String value representing the Dog’s type. This
can either be “retriever”, “terrier”, “husky”, or “mutt”, and default
value is “retriever”.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must include
all the inherited values in their specified order first and then follow
the order of this class’s instance variables given, so first one is
first, second is second, etc This must also check for valid values
before they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of
Domestic Dog and only returns true if all of the instance variables
match. For name and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called Wolf with the following: (5pts)
- Cat must inherit from type Dog
- Instance Variables
- Pack Leader Name: a non-null String value representing
the wolf’s pack leader’s name. The default value is “none”.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Parameterized: The order of the parameters must include
all the inherited values in their specified order first and then follow
the order of this class’s instance variables given, so first one is
first, second is second, etc This must also check for valid values
before they are set.
- Methods
- Accessors and Mutators for the instance variables
- Make sure in the mutators check for valid values named
in the “Instance Variables” Section.
- If the value that is being set is not valid, then set
the instance variable to its default value.
- Equals: This method takes in another instance of Wolf
and only returns true if all of the instance variables match. For name
and type case should be ignored.
- ToString: This method returns a string with all of the
instance variable values concatenated together.
- All the above must apply for full credit.
- Write a class called AnimalCollection with the
following: (20pts)
- Instance Variables
- Animals: This is an array of type Animal which has a
default size of 100.
- Class Constants
- DEF_SIZE: The default size of the array which is 100.
- Constructors
- Default: Must set all instance variables to their
default values defined in the “Instance Variable” sections.
- Methods
- Add Animal: Adds an Animal (Animal, Dog, Cat, House
Cat, Leopard, Domestic Dog, Wolf) to the collection. If the collection
is full, then nothing should happen.
- Remove Animal: Removes an Animal whose data matches an animal
in the array of Animals. If the Animal is not found, then nothing
happens.
- Print Animals: Prints all the animal data to the
console.
- All the above must apply for full credit.
- Write a class called AnimalCollectionFE with the
following: (20pts)
- Static Class Variables
- Keyboard: A static variable of type Scanner who reads
information from the console.
- Animal Collection (aCollection): A static variable of
type AnimalCollection that is constructed using its default
constructor.
- Static Methods
- Main Method: The entry point of the software which
provides access to Animal Collection’s functionality. It also provides
a means to create instances of each animal to either be added or
removed from the collection. After every operation (Add or Remove) it
must print all animals in the collection.
- Other Static Helper Methods: While not strictly
required, it is strongly recommended to organize the functionality of
collecting data (such as the name, weight, mood, and type of a House
Cat) into other separate static methods.
- All the above must apply for full credit.
- Coding Style. (10pts)
- Code functionality organized within multiple methods
other than the main method, and methods organized within multiple classes
where appropriate. (5pts)
- Readable Code. (5pts)
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements
within the body of a class, a method, a branching statement, a loop
statement, etc.
- All the above must apply for full credit.
- Comments. (10pts)
- Your name in every file. (5pts)
- At least 5 meaningful comments in addition to your name.
These must describe the function of the code it is near. (5pts)
Example Dialog:
*The following Example Dialog demonstrates the interactions
between a user and ONE possible implementation of the required software’s
front-end / user interface. The software’s front-end / user interface may
be implemented in MANY different ways and will receive full credit as
long as it meets the most minimal of the above requirements. While you may use
the example dialog as a guide, it is strongly encouraged to create the
front-end / user interface in your own way. *
Key
|
Unhighlighted
Text
|
Program’s
Output
|
Highlighted Text
|
User’s Input
|
Welcome to the Animal Collection!
Enter 1. To Add an Animal.
Enter 2. To Remove an Animal.
Enter 9. To Quit.
1
Enter the kind of Animal.
1. For Animal
2. For Cat
3. For Dog
4. For House Cat
5. For Leopard
6. For Domestic Dog
7. For Wolf
4
Enter the Animal's Name
Mr. Flufferkins
Enter the Animal's Weight
8.6
Enter the Cat's mood. "sleepy", "playful",
"hungry"
playful
Enter the House Cat's Type. "short hair",
"ragdoll", "sphinx", "scottish fold"
ragdoll
----------------------
Name : Mr. Flufferkins
Weight: 8.6
Mood: playful
Type: ragdoll
----------------------
Enter 1. To Add an Animal.
Enter 2. To Remove an Animal.
Enter 9. To Quit.
1
Enter the kind of Animal.
1. For Animal
2. For Cat
3. For Dog
4. For House Cat
5. For Leopard
6. For Domestic Dog
7. For Wolf
6
Enter the Animal's Name
Rufus
Enter the Animal's Weight
20
Enter the Dog's Energy Level. Between 1 and 100.
99
Enter the Domestic Dog's Type. "retriever",
"terrier", "husky", "mutt"
mutt
----------------------
Name : Mr. Flufferkins
Weight: 8.6
Mood: playful
Type: ragdoll
----------------------
----------------------
Name : Rufus
Weight: 20.0
Energy Level: 99
Type: mutt
----------------------
Enter 1. To Add an Animal.
Enter 2. To Remove an Animal.
Enter 9. To Quit.
1
Enter the kind of Animal.
1. For Animal
2. For Cat
3. For Dog
4. For House Cat
5. For Leopard
6. For Domestic Dog
7. For Wolf
1
Enter the Animal's Name
asdf
Enter the Animal's Weight
-4
----------------------
Name : Mr. Flufferkins
Weight: 8.6
Mood: playful
Type: ragdoll
----------------------
----------------------
Name : Rufus
Weight: 20.0
Energy Level: 99
Type: mutt
----------------------
----------------------
Name : asdf
Weight: 0.0
----------------------
Enter 1. To Add an Animal.
Enter 2. To Remove an Animal.
Enter 9. To Quit.
2
Enter the kind of Animal.
1. For Animal
2. For Cat
3. For Dog
4. For House Cat
5. For Leopard
6. For Domestic Dog
7. For Wolf
1
Enter the Animal's Name
asdf
Enter the Animal's Weight
0
----------------------
Name : Mr. Flufferkins
Weight: 8.6
Mood: playful
Type: ragdoll
----------------------
----------------------
Name : Rufus
Weight: 20.0
Energy Level: 99
Type: mutt
----------------------
Enter 1. To Add an Animal.
Enter 2. To Remove an Animal.
Enter 9. To Quit.
1
Enter the kind of Animal.
1. For Animal
2. For Cat
3. For Dog
4. For House Cat
5. For Leopard
6. For Domestic Dog
7. For Wolf
5
Enter the Animal's Name
Fred
Enter the Animal's Weight
120
Enter the Cat's mood. "sleepy", "playful",
"hungry"
hungry
Enter the Leopard's Spots. Greater than or equal to 1.
50
----------------------
Name : Mr. Flufferkins
Weight: 8.6
Mood: playful
Type: ragdoll
----------------------
----------------------
Name : Rufus
Weight: 20.0
Energy Level: 99
Type: mutt
----------------------
----------------------
Name : Fred
Weight: 120.0
Mood: hungry
Number of Spots: 50
----------------------
Enter 1. To Add an Animal.
Enter 2. To Remove an Animal.
Enter 9. To Quit.
9
----------------------
Name : Mr. Flufferkins
Weight: 8.6
Mood: playful
Type: ragdoll
----------------------
----------------------
Name : Rufus
Weight: 20.0
Energy Level: 99
Type: mutt
----------------------
----------------------
Name : Fred
Weight: 120.0
Mood: hungry
Number of Spots: 50
----------------------
Goodbye!
Finally:
- Upload the source code file or files (.JAVA File
Extension) to Homework07
- To Blackboard
- Following these instructions.
- If there are problems, then let the instructor know as
soon as possible.